Reinforcement Learning: A Comprehensive Overview
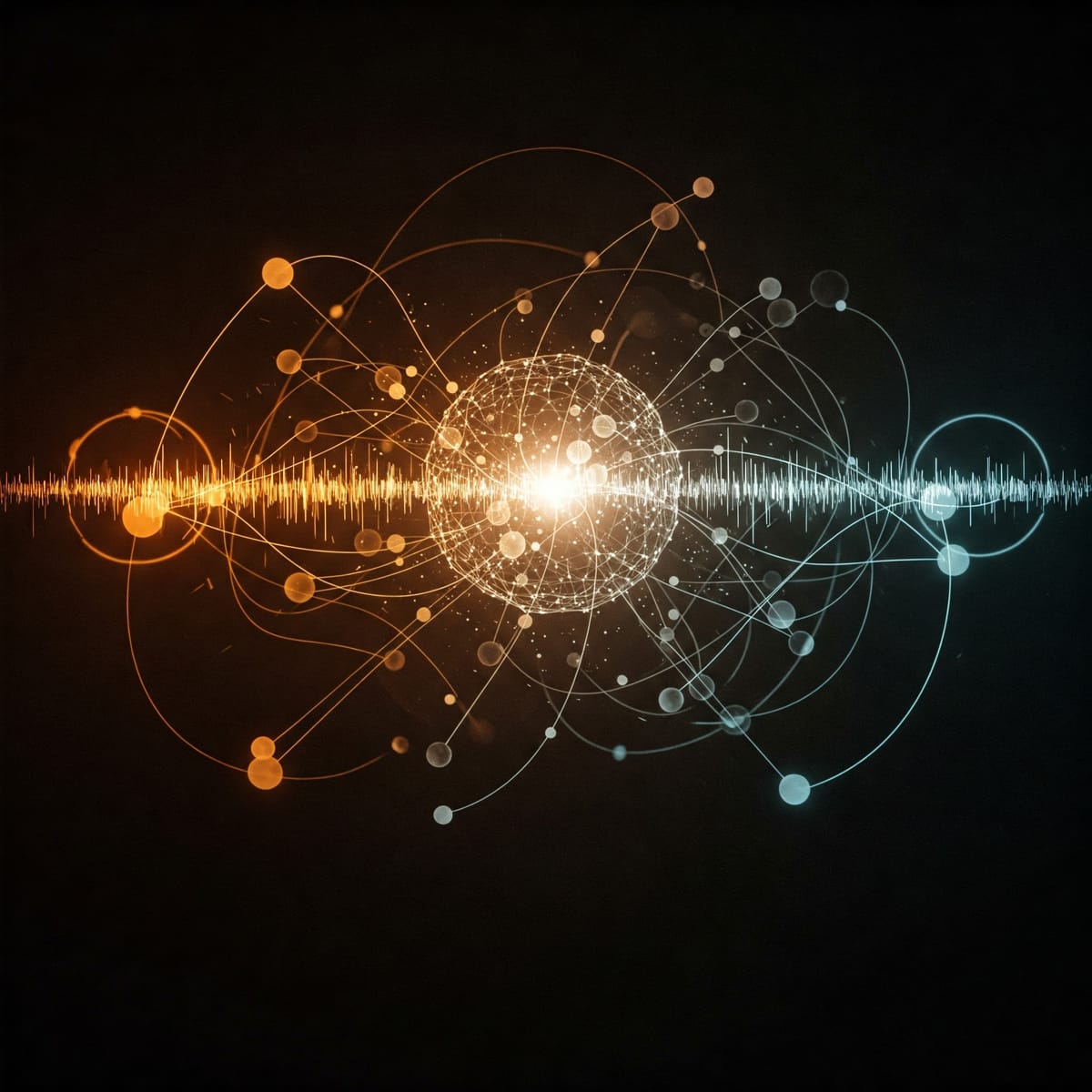
Reinforcement Learning (RL) is a subfield of machine learning where an agent learns to make decisions by interacting with an environment. The agent's goal is to maximize a cumulative reward signal, learning through trial and error. Unlike supervised learning, RL doesn't require labeled data; instead, the agent learns from the consequences of its actions. This makes RL particularly well-suited for problems involving sequential decision-making, such as robotics, game playing, resource management, and control systems.
1. Foundational Concepts and Early Stages
The roots of RL lie in optimal control theory and the study of animal learning. The core concepts that underpin modern RL were established in the mid-20th century:
- Markov Decision Process (MDP): The MDP provides a mathematical framework for modeling sequential decision-making problems. An MDP is defined by:The agent's objective is to find an *optimal policy (π*)**, a mapping from states to actions (or probability distributions over actions) that maximizes the expected cumulative reward (return, Gt), often defined as the discounted sum of future rewards:Gt = Rt+1 + γRt+2 + γ²Rt+3 + ...Source: Bellman, R. (1957). Dynamic Programming. Princeton University Press. Reference: Sutton, R. S., & Barto, A. G. (2018). Reinforcement Learning: An Introduction (2nd ed.). MIT Press. (This is the primary reference for most of the concepts below).
- States (S): A set of possible situations or configurations the environment can be in.
- Actions (A): A set of possible actions the agent can take.
- Transition Probabilities (P): The probability of transitioning from one state to another, given an action: P(s'|s, a). This describes the environment's dynamics.
- Reward Function (R): A function that assigns a numerical reward to each state transition: R(s, a, s'). This represents the immediate feedback the agent receives.
- Discount Factor (γ): A value between 0 and 1 that determines the present value of future rewards. A lower γ prioritizes immediate rewards, while a higher γ considers long-term rewards more heavily.
- Bellman Equations: These equations are fundamental to understanding and solving MDPs. They define the relationship between the value of a state (or state-action pair) and the values of its successor states.The Bellman equations express these value functions recursively:
- State-Value Function (Vπ(s)): The expected return starting from state s and following policy π.Vπ(s) = Eπ[Gt | St = s]
- Action-Value Function (Qπ(s, a)): The expected return starting from state s, taking action a, and then following policy π.Qπ(s, a) = Eπ[Gt | St = s, At = a]
- Bellman Equation for Vπ(s): Vπ(s) = Σa π(a|s) Σs' P(s'|s, a) [R(s, a, s') + γVπ(s')]
- Bellman Equation for Qπ(s, a): Qπ(s, a) = Σs' P(s'|s, a) [R(s, a, s') + γΣa' π(a'|s') Qπ(s', a')]
- Bellman Optimality Equations: These equations define the optimal value functions (V*(s) and Q*(s, a)), which represent the maximum achievable value for each state or state-action pair:V*(s) = max_a Σs' P(s'|s, a) [R(s, a, s') + γV*(s')] Q*(s, a) = Σs' P(s'|s, a) [R(s, a, s') + γ max_a' Q*(s', a')]
2. Tabular Model-Based Algorithms: Dynamic Programming (DP)
Dynamic Programming (DP) methods provide a way to solve MDPs when the model of the environment (transition probabilities and reward function) is known. They use the Bellman equations to iteratively improve value function estimates until convergence.
- Policy Iteration: This algorithm consists of two alternating steps:These two steps are repeated until the policy no longer changes, indicating convergence to the optimal policy.
- Policy Evaluation: Given a policy π, compute the state-value function Vπ(s) for all states. This is typically done iteratively, using the Bellman equation for Vπ(s) as an update rule until convergence.
- Policy Improvement: Create a new policy π' that is greedy with respect to the current value function Vπ(s). That is, for each state s, choose the action a that maximizes the expected return according to the Bellman equation. π'(s) = argmax_a Σs' P(s'|s, a) [R(s, a, s') + γVπ(s')].
- Value Iteration: This algorithm directly computes the optimal value function V*(s) by iteratively applying the Bellman optimality equation as an update rule:V(s) ← max_a Σs' P(s'|s, a) [R(s, a, s') + γV(s')]Once V*(s) converges, the optimal policy can be extracted by choosing the action that maximizes the right-hand side of the Bellman optimality equation for each state.
Code Example (Value Iteration in Python):
Python
import numpy as np
def value_iteration(env, gamma=0.99, theta=1e-6):
"""
Performs value iteration on a given environment.
Args:
env: An environment object with attributes:
- nS: Number of states.
- nA: Number of actions.
- P: Transition probabilities (a dictionary of dictionaries).
P[s][a] is a list of (prob, next_state, reward, done) tuples.
gamma: Discount factor.
theta: Convergence threshold.
Returns:
V: Optimal state-value function (a numpy array).
policy: Optimal policy (a numpy array).
"""
V = np.zeros(env.nS)
policy = np.zeros(env.nS, dtype=int)
while True:
delta = 0
for s in range(env.nS):
v = V[s]
q_values = []
for a in range(env.nA):
q_value = 0
for prob, next_state, reward, done in env.P[s][a]:
q_value += prob * (reward + gamma * V[next_state] * (1 - done))
q_values.append(q_value)
V[s] = max(q_values)
delta = max(delta, abs(v - V[s]))
if delta < theta:
break
# Extract policy
for s in range(env.nS):
q_values = []
for a in range(env.nA):
q_value = 0
for prob, next_state, reward, done in env.P[s][a]:
q_value += prob * (reward + gamma * V[next_state] * (1 - done))
q_values.append(q_value)
policy[s] = np.argmax(q_values)
return V, policy
# Example usage (assuming you have a defined 'env' object)
# V, policy = value_iteration(env)
# print("Optimal Value Function:", V)
# print("Optimal Policy:", policy)
3. Tabular Model-Free Algorithms
Model-free algorithms learn directly from experience without requiring knowledge of the environment's dynamics. This is crucial when the model is unknown or too complex to represent.
- Monte Carlo (MC) Methods: These methods learn from complete episodes of experience. They estimate value functions by averaging the returns observed after visiting a state (or state-action pair).
- First-Visit MC: Averages the returns only for the first visit to a state in each episode.
- Every-Visit MC: Averages the returns for every visit to a state in each episode.
- MC Policy Iteration: Similar to policy iteration in DP, but uses MC methods to estimate the action-value function Qπ(s, a) for the current policy.
- Off-Policy MC Control: Learns about an optimal policy (target policy) while following a different policy (behavior policy). Importance Sampling is used to weight the returns from the behavior policy to estimate the returns for the target policy.
- Temporal Difference (TD) Learning: TD methods combine aspects of both DP and MC. They learn from incomplete episodes, updating value estimates based on the difference between predicted and actual rewards.
- TD(0): The most basic TD method. It updates the value function based on the immediate reward and the estimated value of the next state. The update rule is:V(St) ← V(St) + α[Rt+1 + γV(St+1) - V(St)]where α is the learning rate, and the term in brackets is the TD error (δt).
- TD(0)-Replay: TD(0) is enhanced by dynamically updating the weight parameter.
- TD(λ): Generalizes TD(0) by using eligibility traces. λ is a parameter between 0 and 1 that determines the weighting of n-step returns. Eligibility traces keep track of how much "credit" each state deserves for a received reward.
- N-step TD Prediction: Updates value functions based on rewards received over n steps and the estimated value of the state after n steps. This can reduce variance and speed up learning compared to TD(0).
- N-step Off-Policy Prediction: Extends N-step TD to the off-policy setting, using importance sampling ratios to account for the difference between the target and behavior policies.
- Q-learning: A landmark off-policy TD control algorithm. It learns the optimal action-value function Q*(s, a) directly, without needing to estimate a policy explicitly. The update rule is: Q(St, At) ← Q(St, At) + α[Rt+1 + γ max_a Q(St+1, a) - Q(St, At)]
- Double Q-learning: Addresses the overestimation bias in Q-learning by using two separate Q-value estimators. One is used to select the action, and the other is used to evaluate the action.
- SARSA (State-Action-Reward-State-Action): An on-policy TD control algorithm. Unlike Q-learning, which uses the maximum Q-value of the next state, SARSA uses the Q-value of the next state and the action actually taken according to the current policy. The update rule is:Q(St, At) ← Q(St, At) + α[Rt+1 + γQ(St+1, At+1) - Q(St, At)]
- Expected SARSA: Instead of sampling the next action (At+1) as in SARSA, Expected SARSA uses the expected value of the next state-action pairs, weighted by the current policy's probabilities. This can reduce variance.
- N-step SARSA: SARSA can be extended by incorporating a sequence of n actions.
- Hybrid On-Policy/Off-Policy N-step Methods: Combine elements of on-policy (like SARSA) and off-policy (like Q-learning) methods with N-step look-ahead.
4. Approximation Model-Free Algorithms (Deep RL)
When the state space is very large or continuous, tabular methods become impractical. Function approximation is used to generalize from seen states to unseen states. Deep neural networks have become a powerful tool for function approximation in RL.
- Deep Q-learning (DQN): Uses a deep neural network to approximate the action-value function Q(s, a; θ), where θ represents the network's weights. DQN introduced two key innovations to stabilize learning:Source: Mnih, V., Kavukcuoglu, K., Silver, D., et al. (2015). Human-level control through deep reinforcement learning. Nature, 518(7540), 529–533.
- Experience Replay: Stores past experiences (transitions) in a replay buffer and samples mini-batches from this buffer to update the network. This breaks correlations between consecutive updates and improves data efficiency.
- Target Network: Uses a separate, slowly updated network to compute the target Q-values in the update rule. This reduces oscillations and instability.
- Double Deep Q-learning (DDQN): Combines Double Q-learning with DQN to mitigate the overestimation bias of Q-values, further improving performance.
5. Tabular Model-Based Algorithms - Model-Based Planning
Model-based methods learn or are given a model of the environment and use it for planning.
- Model-based Planning
- Prioritized Sweeping: Focuses updates on states where the value function is likely to change the most.
- Monte Carlo Tree Search (MCTS): A powerful search algorithm that combines tree search with Monte Carlo simulations. It's particularly effective in games with large state spaces (e.g., Go). MCTS involves four main steps:Source: Browne, C. B., Powley, E., Whitehouse, D., et al. (2012). A survey of Monte Carlo tree search methods. IEEE Transactions on Computational Intelligence and AI in Games, 4(1), 1–43.
- Selection: Traverse the tree from the root node, selecting actions based on a selection strategy (e.g., Upper Confidence Bound applied to Trees - UCT).
- Expansion: If a leaf node is reached and it's not a terminal state, expand the tree by adding one or more child nodes.
- Simulation (Rollout): From the newly expanded node, simulate a rollout to the end of the episode using a default policy (often a random policy).
- Backpropagation: Update the values of the nodes along the path traversed during selection, based on the result of the rollout.
- Dyna-Q: Integrates learning and planning. It learns a model from real experience and uses it to generate simulated experiences, which are then used to update the value function and policy using Q-learning.
6. Policy Gradient Methods
Policy gradient methods directly optimize the policy parameters θ to maximize the expected return. They work by estimating the gradient of the expected return with respect to θ and updating the policy in the direction of the gradient.
- REINFORCE: A fundamental policy gradient algorithm. It uses Monte Carlo sampling to estimate the gradient of the expected return. The update rule is:θ ← θ + α ∇θ log πθ(At|St) Gtwhere α is the learning rate, πθ(At|St) is the policy parameterized by θ, and Gt is the return from time step t.
- Trust Region Policy Optimization (TRPO): Improves the stability of policy gradient methods by constraining the change in the policy at each update. It uses the KL divergence between the old and new policies as a constraint.Source: Schulman, J., Levine, S., Abbeel, P., Jordan, M., & Moritz, P. (2015). Trust region policy optimization. In International Conference on Machine Learning (pp. 1889-1897).
- Proximal Policy Optimization (PPO): A simpler and more efficient algorithm than TRPO, while still maintaining stability. It uses a clipped surrogate objective function to limit the policy update size. Source: Schulman, J., Wolski, F., Dhariwal, P., Radford, A., & Klimov, O. (2017). Proximal policy optimization algorithms. arXiv preprint arXiv:1707.06347.
7. Actor-Critic Methods
Actor-critic methods combine the advantages of both value-based and policy-based methods. They use an actor (the policy) and a critic (a value function). The critic estimates the value function, which is used to guide the updates of the actor.
- Actor-Critic Methods (General)
- Advantage Actor-Critic (A2C): A synchronous version of the actor-critic method. Multiple agents collect experience in parallel, and the updates are performed synchronously. This improves stability and data efficiency.
- Deterministic Policy Gradient (DPG): is an actor-critic method for continuous action spaces.
- Deep Deterministic Policy Gradient (DDPG): Extends DPG to use deep neural networks for both the actor and critic. It incorporates techniques from DQN (experience replay and target networks) for stability in continuous action spaces.Source: Lillicrap, T. P., Hunt, J. J., Pritzel, A., Heess, N., Erez, T., Tassa, Y., ... & Wierstra, D. (2015). Continuous control with deep reinforcement learning. arXiv preprint arXiv:1509.02971.
- Twin Delayed Deep Deterministic Policy Gradient (TD3): An enhancement of DDPG that addresses the issue of overestimation bias in the critic. TD3 introduces three key improvements:Source: Fujimoto, S., Hoof, H., & Meger, D. (2018). Addressing function approximation error in actor-critic methods. In International Conference on Machine Learning (pp. 1587-1596).
8. Challenges and Future Directions in Reinforcement Learning
While RL has achieved impressive results, several challenges remain:
- Sample Efficiency: Many RL algorithms require a large amount of experience to learn effectively. This can be a significant bottleneck, especially in real-world applications where data collection is expensive or time-consuming. Research areas addressing this include:
- Model-Based RL: Learning a model of the environment can significantly reduce the amount of real-world interaction needed.
- Transfer Learning: Leveraging knowledge learned from previous tasks to accelerate learning on new tasks.
- Meta-Learning: Learning to learn, enabling agents to quickly adapt to new environments with minimal experience.
- Off-Policy Learning: Making better use of existing data generated by different policies.
- Exploration-Exploitation Dilemma: Finding the right balance between exploring the environment to discover new information and exploiting the current knowledge to maximize rewards is a fundamental challenge. Methods like ε-greedy, Upper Confidence Bounds (UCB), and Thompson Sampling are used to address this.
- Safety and Robustness: Ensuring that RL agents behave safely and reliably in real-world environments is critical. This is particularly important in safety-critical applications like robotics and autonomous driving. Research areas include:
- Safe Reinforcement Learning: Developing algorithms that explicitly consider safety constraints during learning.
- Adversarial Robustness: Training agents that are robust to adversarial attacks or perturbations in the environment.
- Explainable Reinforcement Learning (XRL): Making the decision-making process of RL agents more understandable and interpretable.
- Generalization: RL agents often struggle to generalize to new environments or situations that differ from their training environment. Improving generalization is an active area of research.
- High-Dimensional and Continuous State and Action Spaces: Dealing with complex environments with high-dimensional or continuous state and action spaces remains a challenge. Deep RL has made significant progress, but further improvements are needed.
- Partial Observability: Many real-world problems involve partial observability, where the agent doesn't have complete information about the environment's state. Partially Observable Markov Decision Processes (POMDPs) provide a framework for these problems, but solving POMDPs is generally much harder than solving MDPs.
- Reward Shaping/Specification: Designing a good reward function that accurately reflects the desired behavior can be difficult and may lead to unintended consequences if not done carefully. Inverse Reinforcement Learning (IRL) attempts to learn a reward function from expert demonstrations.
9. Conclusion
Reinforcement learning has emerged as a powerful framework for learning sequential decision-making in complex environments. From its foundations in dynamic programming and optimal control, RL has advanced rapidly, particularly with the advent of deep learning. While significant challenges remain, ongoing research continues to push the boundaries of what's possible, paving the way for increasingly intelligent and autonomous agents capable of solving real-world problems. The field is continuously evolving, with new algorithms and techniques being developed regularly. The combination of theoretical advances, increased computational power, and the availability of large datasets is driving rapid progress in RL, making it one of the most exciting and promising areas of artificial intelligence.
10. Expanded References and Resources
- Books:
- Sutton, R. S., & Barto, A. G. (2018). Reinforcement Learning: An Introduction (2nd ed.). MIT Press. (The standard textbook, available online for free).
- Bertsekas, D. P. (2019). Reinforcement Learning and Optimal Control. Athena Scientific. (More mathematically focused).
- Powell, W. B. (2011). Approximate Dynamic Programming: Solving the Curses of Dimensionality (2nd ed.). Wiley.
- Szepesvári, C. (2010). Algorithms for Reinforcement Learning. Morgan & Claypool Publishers.
- Key Papers (already mentioned above, but repeated for completeness):
- Bellman, R. (1957). Dynamic Programming. Princeton University Press.
- Mnih, V., Kavukcuoglu, K., Silver, D., et al. (2015). Human-level control through deep reinforcement learning. Nature, 518(7540), 529–533.
- Schulman, J., Levine, S., Abbeel, P., Jordan, M., & Moritz, P. (2015). Trust region policy optimization. In International Conference on Machine Learning (pp. 1889-1897).
- Schulman, J., Wolski, F., Dhariwal, P., Radford, A., & Klimov, O. (2017). Proximal policy optimization algorithms. arXiv preprint arXiv:1707.06347.
- Lillicrap, T. P., Hunt, J. J., Pritzel, A., Heess, N., Erez, T., Tassa, Y., ... & Wierstra, D. (2015). Continuous control with deep reinforcement learning. arXiv preprint arXiv:1509.02971.
- Fujimoto, S., Hoof, H., & Meger, D. (2018). Addressing function approximation error in actor-critic methods. In International Conference on Machine Learning (pp. 1587-1596).
- Browne, C. B., Powley, E., Whitehouse, D., et al. (2012). A survey of Monte Carlo tree search methods. IEEE Transactions on Computational Intelligence and AI in Games, 4(1), 1–43.
- Online Courses and Tutorials:
- David Silver's UCL Reinforcement Learning Course (YouTube):https://www.youtube.com/playlist?list=PLqYmG7hTraZDM-OYHWgPebj2MfCFzFObQ
- DeepMind x UCL | Deep Learning Lectures | 2021 (YouTube):https://www.youtube.com/playlist?list=PLqYmG7hTraZBKeNJ-JE_eyJHZ7XgBoAyb
- Spinning Up in Deep RL (OpenAI):https://spinningup.openai.com/(Excellent introduction and resources)
- Coursera Reinforcement Learning Specialization (University of Alberta): A series of courses covering various RL topics.
- fast.ai (Practical Deep Learning for Coders): Includes sections on reinforcement learning.
- Software Libraries:
- TensorFlow Agents: A library for reinforcement learning in TensorFlow.
- PyTorch: Widely used for deep learning, and has excellent support for RL.
- Stable Baselines3: A set of reliable implementations of reinforcement learning algorithms in PyTorch.
- RLLib (Ray): A scalable library for reinforcement learning.
- OpenAI Gym: A toolkit for developing and comparing reinforcement learning algorithms. Provides a wide variety of environments.
- Clipped Double Q-Learning: Uses two critic networks (like Double Q-learning) and takes the minimum of the two Q-value estimates to reduce overestimation.
- Delayed Policy Updates: Updates the policy (actor) less frequently than the critic. This helps to reduce the accumulation of errors from the critic in the policy updates.
- Target Policy Smoothing: Adds noise to the target action when computing the target Q-value. This smooths the value function and makes learning more stable.
Comments ()