Random Forests: A Powerful and Robust Ensemble Method
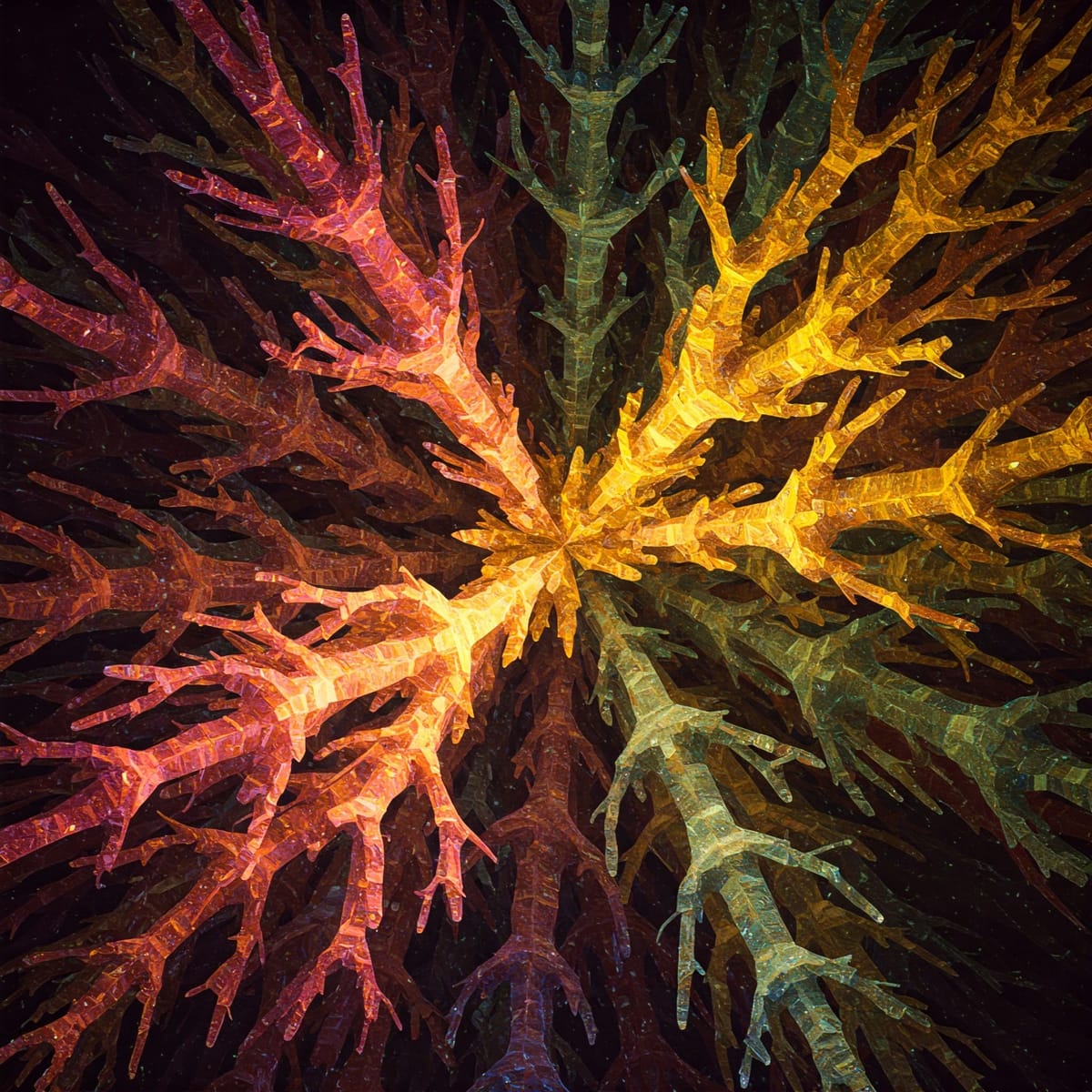
Random Forests have emerged as a cornerstone of modern machine learning, known for their high accuracy, robustness, and ease of use. This article delves into the intricacies of Random Forests, exploring their underlying principles, applications, advantages, limitations, and providing Python code examples to illustrate their practical implementation.
1. Introduction to Random Forests:
A Random Forest is an ensemble learning method that operates by constructing a multitude of decision trees during training and outputs the class that is the mode of the classes (classification) or mean prediction (regression) of the individual trees. In essence, it leverages the wisdom of the crowd – a collection of diverse and independent decision trees – to make more accurate and robust predictions than any single decision tree could achieve on its own.
2. Underlying Principles:
Random Forests build on the foundation of decision trees but introduce randomness in two crucial ways:
- Bagging (Bootstrap Aggregating): Random Forests use bagging to create multiple subsets of the original training data. Each tree is trained on a different bootstrap sample (sampled with replacement) from the original dataset. This introduces variation and reduces the risk of overfitting.
- Random Feature Selection: At each split within a decision tree, only a random subset of the features is considered. Instead of evaluating all possible features for the best split, the algorithm randomly selects m features (where m is typically much smaller than the total number of features, p) and chooses the best split among those m features. This further decorrelates the trees and contributes to the forest's robustness.
3. Algorithm Overview:
- Bootstrap Sampling: Generate n bootstrap samples from the original training data.
- Tree Construction: For each bootstrap sample:
- Build a decision tree.
- At each node in the tree, randomly select m features.
- Find the best split among those m features based on a splitting criterion (e.g., Gini impurity or information gain for classification, mean squared error for regression).
- Grow the tree to its maximum depth (or until a stopping criterion is met, such as a minimum number of samples per leaf). Note: Random Forests often grow trees to their maximum depth without pruning, leveraging the averaging effect of the ensemble to mitigate overfitting.
- Prediction: For a new data point:
- Feed the data point to each of the n trees.
- Aggregate the predictions from each tree. For classification, take the majority vote; for regression, take the average.
4. Applications:
Random Forests are applicable across a wide range of domains:
- Classification:
- Image Recognition: Classifying objects in images (e.g., identifying animals, plants, or vehicles).
- Medical Diagnosis: Predicting diseases based on patient symptoms and medical history.
- Credit Risk Assessment: Determining the likelihood of a loan applicant defaulting.
- Spam Detection: Identifying and filtering spam emails.
- Regression:
- Sales Forecasting: Predicting future sales based on historical data and market trends.
- Stock Price Prediction: Forecasting stock prices based on historical data and market indicators.
- Environmental Modeling: Predicting weather patterns, air quality, or water levels.
- House Price Prediction: Estimating house prices based on location, size, and other features.
- Feature Importance Estimation: Random Forests can be used to estimate the importance of different features in a dataset. This can be valuable for feature selection and gaining insights into the underlying data.
5. Advantages of Random Forests:
- High Accuracy: Often achieves state-of-the-art performance compared to other machine learning algorithms.
- Robustness: Less prone to overfitting due to the averaging effect of multiple trees and the random feature selection process. It handles outliers and missing values relatively well (though imputation may still be beneficial).
- Ease of Use: Requires minimal data preprocessing and hyperparameter tuning. Many implementations provide sensible default values.
- Handles High-Dimensional Data: Effectively handles datasets with a large number of features.
- Feature Importance Estimation: Provides a measure of the importance of each feature in the model.
- Versatile: Can be used for both classification and regression tasks.
- Parallelization: The construction of individual trees can be easily parallelized, leading to faster training times.
6. Limitations of Random Forests:
- Interpretability: More difficult to interpret than single decision trees. While feature importance can provide some insights, understanding the specific relationships between features and predictions can be challenging.
- Computational Cost: Can be computationally expensive to train, especially with a large number of trees and a large dataset.
- Bias towards Features with Many Categories: When dealing with categorical features, Random Forests can be biased towards features with more categories. This is because features with more categories have more opportunities to improve the splitting criterion.
- Extrapolation Limitations: Random Forests don't extrapolate well beyond the range of values seen in the training data. In regression tasks, predictions outside the training range may be unreliable.
7. Python Code Examples:
Example 1: Classification with scikit-learn
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
from sklearn.metrics import accuracy_score
# Load the Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create a Random Forest Classifier
rf_classifier = RandomForestClassifier(n_estimators=100, random_state=42) # n_estimators: number of trees
# Train the model
rf_classifier.fit(X_train, y_train)
# Make predictions
y_pred = rf_classifier.predict(X_test)
# Evaluate the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
# Feature Importance
feature_importances = rf_classifier.feature_importances_
print("Feature Importances:", feature_importances)
Example 2: Regression with scikit-learn
from sklearn.ensemble import RandomForestRegressor
from sklearn.model_selection import train_test_split
from sklearn.datasets import make_regression
from sklearn.metrics import mean_squared_error
# Generate a synthetic regression dataset
X, y = make_regression(n_samples=100, n_features=4, random_state=42)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create a Random Forest Regressor
rf_regressor = RandomForestRegressor(n_estimators=100, random_state=42)
# Train the model
rf_regressor.fit(X_train, y_train)
# Make predictions
y_pred = rf_regressor.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
# Feature Importance
feature_importances = rf_regressor.feature_importances_
print("Feature Importances:", feature_importances)
Example 3: Hyperparameter Tuning with GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.datasets import load_iris
# Load the Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Define the parameter grid
param_grid = {
'n_estimators': [50, 100, 200],
'max_depth': [None, 5, 10],
'min_samples_split': [2, 5, 10],
'min_samples_leaf': [1, 2, 4]
}
# Create a Random Forest Classifier
rf_classifier = RandomForestClassifier(random_state=42)
# Create a GridSearchCV object
grid_search = GridSearchCV(estimator=rf_classifier, param_grid=param_grid, cv=3, scoring='accuracy')
# Perform the grid search
grid_search.fit(X_train, y_train)
# Print the best parameters and score
print("Best Parameters:", grid_search.best_params_)
print("Best Score:", grid_search.best_score_)
# Evaluate the model with the best parameters
best_rf = grid_search.best_estimator_
accuracy = best_rf.score(X_test, y_test)
print(f"Accuracy on Test Set with Best Parameters: {accuracy}")
Explanation of Key Parameters in scikit-learn's RandomForestClassifier and RandomForestRegressor:
- n_estimators: The number of trees in the forest. A higher number generally leads to better performance, but also increases training time.
- max_depth: The maximum depth of each tree. None means trees are grown until all leaves are pure or until all leaves contain less than min_samples_split samples. Limiting depth can help prevent overfitting.
- min_samples_split: The minimum number of samples required to split an internal node.
- min_samples_leaf: The minimum number of samples required to be at a leaf node.
- max_features: The number of features to consider when looking for the best split. 'auto' (or 'sqrt') uses the square root of the number of features. 'log2' uses the base 2 logarithm. None uses all features (which is not typically recommended).
- bootstrap: Whether bootstrap samples are used when building trees. Set to False to use the whole dataset to build each tree.
- random_state: A seed for the random number generator. This ensures reproducibility of the results.
8. References and Further Reading:
- Breiman, L. (2001). Random forests. Machine Learning, 45(1), 5-32. https://doi.org/10.1023/A:1010933404324
- Elements of Statistical Learning (Hastie, Tibshirani, and Friedman): A comprehensive textbook covering statistical learning methods, including Random Forests.
- Scikit-learn Documentation: https://scikit-learn.org/stable/modules/generated/sklearn.ensemble.RandomForestClassifier.html and https://scikit-learn.org/stable/modules/generated/sklearn.ensemble.RandomForestRegressor.html
- Kaggle: Explore Kaggle competitions and notebooks to see real-world applications of Random Forests.
9. Conclusion:
Random Forests are a powerful and versatile machine learning algorithm, well-suited for a wide range of classification and regression problems. Their robustness, ease of use, and ability to handle high-dimensional data make them a valuable tool for any data scientist or machine learning practitioner. While they have some limitations, such as lower interpretability and potential computational cost, the advantages often outweigh these drawbacks, making them a reliable and effective choice for many applications. By understanding the underlying principles and best practices, you can effectively leverage Random Forests to build accurate and robust predictive models.
Comments ()