Cross-Validation: Building Trustworthy Machine Learning Models Through Robust Evaluation
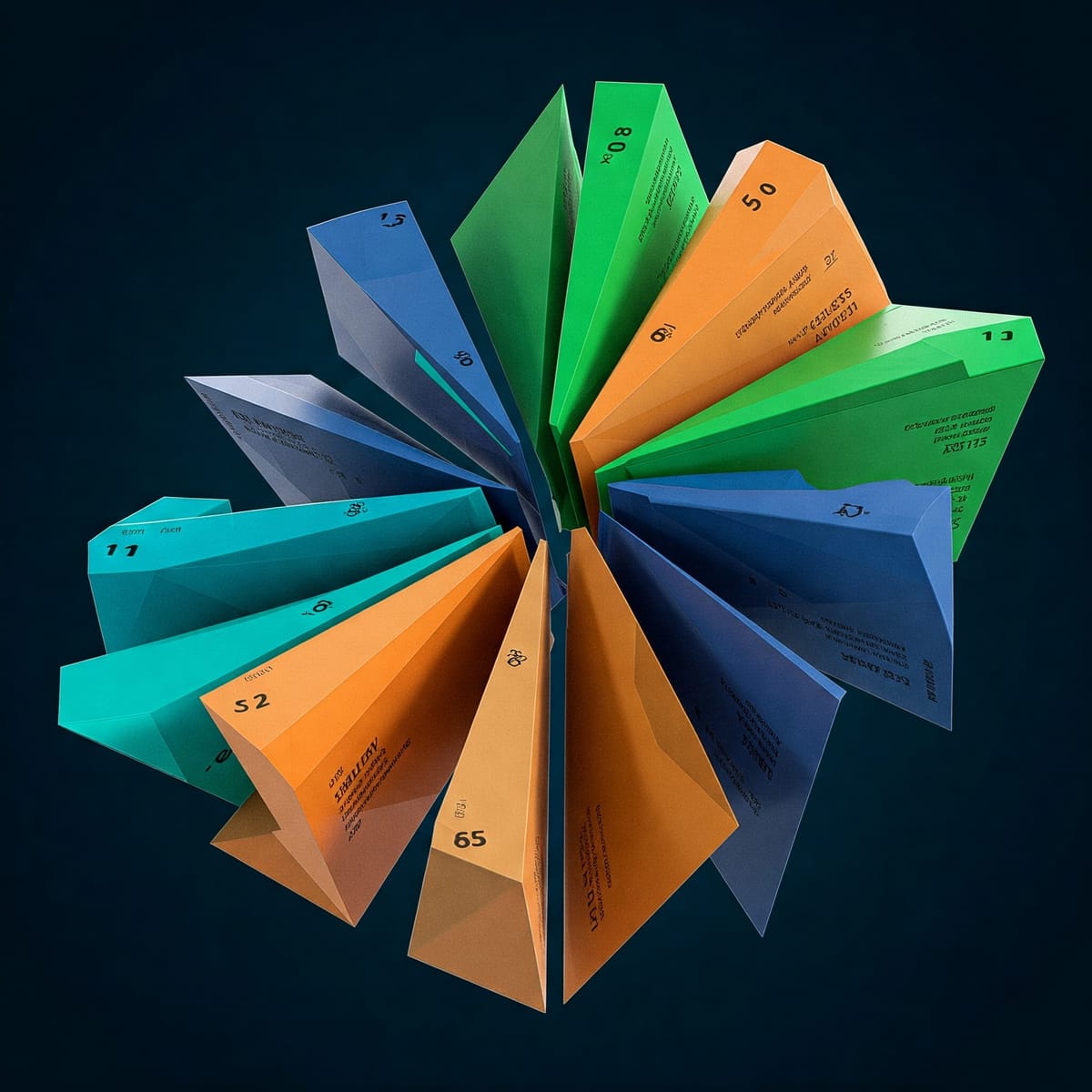
In machine learning, the ultimate goal is to build models that generalize well to unseen data. We want our models to perform accurately not just on the data they were trained on, but also on new, real-world data. Simply evaluating a model on the same data it was trained on can lead to overly optimistic performance estimates and the dreaded problem of overfitting. This is where cross-validation comes in. Cross-validation is a powerful set of techniques used to assess how well a model will generalize to an independent dataset. It provides a more reliable estimate of model performance than a single train/test split, making it an essential part of the machine learning workflow.
1. The Problem with a Single Train/Test Split
The traditional approach of splitting data into a single training set and a single test set has a significant drawback: the performance estimate can be highly dependent on which data points happen to end up in the training set and which end up in the test set. If, by chance, the test set is particularly "easy" or contains data that is very similar to the training data, the model will appear to perform better than it actually would on truly unseen data. Conversely, if the test set is particularly "hard," the model's performance will be underestimated. This variability makes it difficult to compare different models or hyperparameter settings reliably.
2. What is Cross-Validation?
Cross-validation addresses this problem by systematically partitioning the data into multiple subsets and iteratively training and evaluating the model on different combinations of these subsets. The core idea is to use all of the available data for both training and testing, but in a way that avoids testing on the same data used for training. This provides a more robust and less biased estimate of the model's generalization performance.
3. K-Fold Cross-Validation: The Workhorse
The most common and widely used cross-validation technique is k-fold cross-validation. Here's how it works:
- Partition: The original dataset is randomly partitioned into k equal-sized (or nearly equal-sized) subsets, called "folds."
- Iteration: The following process is repeated k times:
- One of the k folds is held out as the validation set (or "test fold").
- The remaining k-1 folds are combined to form the training set.
- The model is trained on the training set.
- The model is evaluated on the held-out validation set. The performance metric (e.g., accuracy, F1-score, MSE) is recorded.
- Average: After k iterations, you have k different performance scores (one for each fold). The final cross-validation score is the average of these k scores.
Visual Representation:
Imagine k = 5
:
| Fold 1 | Fold 2 | Fold 3 | Fold 4 | Fold 5 |
-----------------------------------------------
| Test | Train | Train | Train | Train | Iteration 1
| Train | Test | Train | Train | Train | Iteration 2
| Train | Train | Test | Train | Train | Iteration 3
| Train | Train | Train | Test | Train | Iteration 4
| Train | Train | Train | Train | Test | Iteration 5
Key Parameters:
- k: The number of folds. Common choices are k = 5 and k = 10. Larger values of k generally lead to lower bias (more data used for training) but higher variance (smaller validation sets, more variability in the performance estimates). Smaller values of k have lower variance but potentially higher bias. There's no universally "best" value; it often depends on the dataset size.
- Shuffling: It's crucial to randomly shuffle the data before partitioning it into folds. This helps ensure that each fold is a representative sample of the overall dataset and avoids any systematic bias due to the original ordering of the data.
Advantages of K-Fold Cross-Validation:
- More Reliable Performance Estimate: Provides a more robust and less biased estimate of the model's generalization performance compared to a single train/test split.
- Uses All Data: All data points are used for both training and validation, making efficient use of limited data.
- Reduces Overfitting: Helps to mitigate overfitting by evaluating the model on multiple independent validation sets.
- Model Comparison: Provides a more reliable way to compare different models or hyperparameter settings.
4. Variations of K-Fold Cross-Validation
- Stratified K-Fold: This is a crucial variation when dealing with imbalanced datasets (where the classes are not equally represented). Stratified k-fold ensures that each fold contains approximately the same proportion of each class as the original dataset. This prevents a situation where, by chance, one fold might contain only samples from a single class, leading to unreliable performance estimates.
- Repeated K-Fold: This involves repeating the k-fold cross-validation process multiple times, with different random shuffles of the data each time. This can further reduce the variance of the performance estimate.
- Leave-One-Out Cross-Validation (LOOCV): This is an extreme case of k-fold cross-validation where k is equal to the number of data points. Each data point is held out as the validation set once, and the model is trained on the remaining n-1 data points. LOOCV has very low bias but can have high variance, especially for large datasets, and is computationally expensive.
- Time Series Cross-Validation: For time-series data, it's crucial to maintain the temporal order. Standard k-fold cross-validation is not appropriate because it would lead to "future" data being used to predict "past" data. Time series cross-validation techniques, such as rolling-origin cross-validation or forward chaining, ensure that the validation set always comes after the training set in time.
- Rolling Origin: The training set consists of observations from time 1 to t, and the validation set consists of observations from time t+1. The origin (the starting point of the training set) is then rolled forward in time.
- Forward Chaining: A series of training sets are created, each adding one more time step. The validation set always follows the training set.
- Group K-Fold: If there are groups within the data that should not be split between folds this allows specifying which groups should stay together.
5. Implementing K-Fold Cross-Validation (Python Example with Scikit-learn)
from sklearn.model_selection import KFold, cross_val_score, StratifiedKFold
from sklearn.linear_model import LogisticRegression # Example model
from sklearn.datasets import make_classification
# Generate a synthetic dataset
X, y = make_classification(n_samples=100, n_features=20, random_state=42)
# Create a model (Logistic Regression in this example)
model = LogisticRegression()
# --- K-Fold Cross-Validation ---
kf = KFold(n_splits=5, shuffle=True, random_state=42) # 5 folds, shuffled
cv_scores = cross_val_score(model, X, y, cv=kf, scoring='accuracy') # scoring can be changed
print("K-Fold Cross-Validation Scores:", cv_scores)
print("Mean K-Fold CV Score:", cv_scores.mean())
print("Standard Deviation of K-Fold CV Score", cv_scores.std())
# --- Stratified K-Fold Cross-Validation ---
skf = StratifiedKFold(n_splits=5, shuffle=True, random_state=42)
cv_scores_stratified = cross_val_score(model, X, y, cv=skf, scoring='accuracy')
print("\nStratified K-Fold Cross-Validation Scores:", cv_scores_stratified)
print("Mean Stratified K-Fold CV Score:", cv_scores_stratified.mean())
print("Standard Deviation of Stratified K-Fold CV Score:", cv_scores_stratified.std())
6. Limitations of Cross-Validation
- Computational Cost: Cross-validation can be computationally expensive, especially for large datasets and complex models, as it requires training the model multiple times.
- Data Distribution Assumptions: Cross-validation assumes that the data is independently and identically distributed (i.i.d.). This assumption may not hold for all datasets, particularly time-series data or data with group structures.
- Still needs a Test Set: While cross validation gives a robust estimate of validation performance, a completely separate test set is still recommended for final model evaluation. Cross-validation should be used for model selection and hyperparameter tuning. The test set is kept untouched until the very end.
7. Conclusion
Cross-validation, particularly k-fold cross-validation and its variations, is a fundamental technique for building robust and reliable machine learning models. It provides a more accurate estimate of a model's generalization performance than a single train/test split, helping to prevent overfitting and allowing for more reliable model comparison and hyperparameter tuning. While computationally more expensive, the benefits of cross-validation in terms of model trustworthiness far outweigh the costs in most practical applications. Remember to always combine cross-validation with a final, held-out test set for the ultimate, unbiased evaluation of your chosen model.
References
[1] Kohavi, R. (1995). A study of cross-validation and bootstrap for accuracy estimation and model selection. In Ijcai (Vol. 14, No. 2, pp. 1137-1145).
[2] Hastie, T., Tibshirani, R., & Friedman, J. (2009). The Elements of Statistical Learning: Data Mining, Inference, and Prediction (2nd ed.). Springer.
[3] James, G., Witten, D., Hastie, T., & Tibshirani, R. (2013). An Introduction to Statistical Learning: with Applications in R. Springer.
[4] Arlot, S., & Celisse, A. (2010). A survey of cross-validation procedures for model selection. Statistics surveys, 4, 40-79.
[5] Geisser, S. (1975). The predictive sample reuse method with applications. Journal of the American Statistical Association, 70(350), 320-328.
[6] Stone, M. (1974). Cross-validatory choice and assessment of statistical predictions. Journal of the Royal Statistical Society: Series B (Methodological), 36(2), 111-133.
[7] Bergstra, J., & Bengio, Y. (2012). Random search for hyper-parameter optimization. Journal of Machine Learning Research, 13(Feb), 281-305.
Comments ()